Embark on a journey into the world of C++ early objects with this comprehensive guide, “Starting Out with C++ Early Objects PDF.” Delve into the fundamentals, applications, and best practices of this powerful programming technique, unlocking its potential for efficient and maintainable code.
C++ early objects offer a unique approach to object-oriented programming, enabling developers to create and initialize objects before the program’s main function. This guide provides a thorough understanding of their benefits, types, and effective usage, empowering you to harness their capabilities.
Introduction to C++ Early Objects
C++ early objects are a powerful language feature that allows developers to define and use objects before their class is fully defined. This can be beneficial in several ways, such as improving code organization, maintainability, and performance.
There are two main types of early objects: forward declarations and extern declarations. Forward declarations simply declare the name and type of the object, while extern declarations also specify the memory location of the object.
Creating and Using Early Objects
To create an early object, simply declare it using the forward or extern declaration syntax. For example:
// Forward declaration class MyClass; // Extern declaration extern MyClass myObject;
Once an early object is declared, it can be used like any other object. For example, you can assign values to its members, call its methods, and pass it to functions.
Benefits of Using Early Objects, Starting out with c++ early objects pdf
There are several benefits to using early objects, including:
- Improved code organization:Early objects can help to improve code organization by allowing you to define objects before their class is fully defined. This can make it easier to read and understand your code.
- Increased maintainability:Early objects can also help to increase maintainability by making it easier to change the definition of a class without affecting the code that uses it.
- Enhanced code performance:In some cases, early objects can also enhance code performance by reducing the amount of time it takes to load a class.
Best Practices for Using Early Objects
When using early objects, it is important to follow a few best practices to ensure that your code is efficient and maintainable.
- Use forward declarations whenever possible:Forward declarations are more efficient than extern declarations and they can help to reduce the risk of errors.
- Only use extern declarations when necessary:Extern declarations should only be used when you need to access the memory location of an object.
- Document your early objects:It is important to document your early objects so that other developers can understand how they are used.
Case Studies and Examples
Early objects have been used in a variety of successful C++ projects. One example is the Linux kernel, which uses early objects to define data structures before they are fully defined.
Another example is the Boost library, which uses early objects to define templates before they are fully defined.
Quick FAQs: Starting Out With C++ Early Objects Pdf
What are the advantages of using early objects in C++?
Early objects provide several advantages, including improved code organization, enhanced maintainability, and potential performance gains.
How do I create an early object in C++?
To create an early object in C++, you can use the following syntax: extern object_type object_name = initializer_list;
What are the different types of early objects?
There are two main types of early objects: static early objects and thread-local early objects.
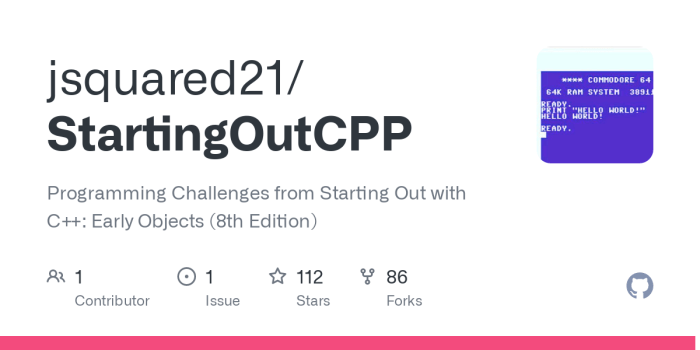